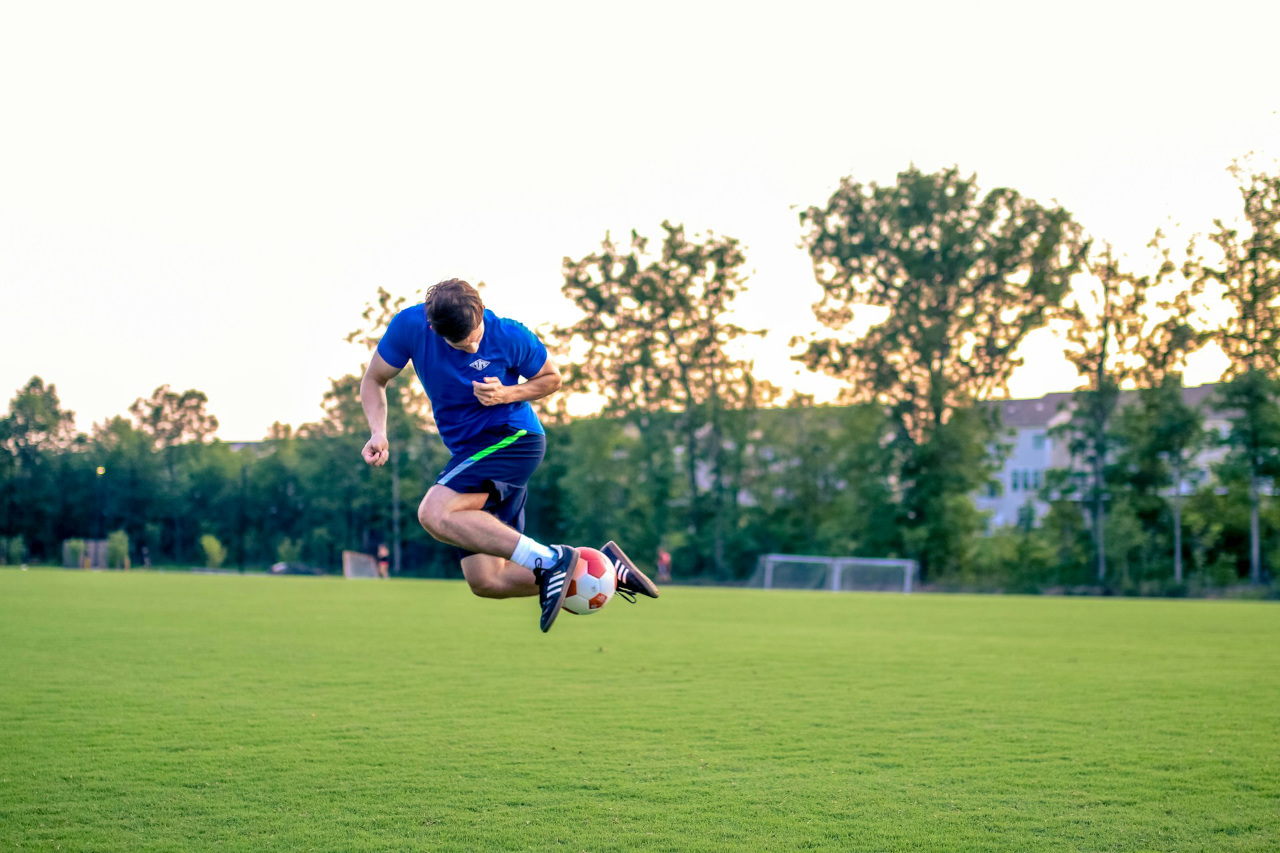
Best Practice for using Entity Framework Core with ASP.NET Core Blazor
Using Entity Framework Core with ASP.NET Core Blazor is not the same as ASP.NET Core. While working MyBot.Chat project, I ran into many random error related to concurrency issue. Microsoft documentation (reference below) provide some suggestion on some good approach to use Entity Framework Core correctly with ASP.NET Core Blazor.
After some trial and error, I picked this below solution that work best. So here is some example code extraced from ShipFast-ASP.NET code base:
public class ProductService : IProductService
{
IDbContextFactory<ApplicationDbContext> contextFactory;
public ProductService(IDbContextFactory<ApplicationDbContext> contextFactory)
{
this.contextFactory = contextFactory;
}
public async Task<bool> UserPaidForProduct(string userId){
using var _context = contextFactory.CreateDbContext();
var product = await _context.Products.Where(x => x.UserId == userId).FirstOrDefaultAsync();
if(product == null || product.PaymentStatus != "paid") return false;
return true;
}
}
The trick is to switch to use and inject IDbContextFactory and in every class and create a new DbContext in every function, and you want to automatic garbage colleciton to discard the DBContext immediately after exiting the function to avoid concurrency issue:
using var _context = contextFactory.CreateDbContext();
Do not worry about the performance of creating DbContext in every function, it should be fast.